This is the quick fix option for Visual Code that helps the user to add source code.
For example, you can add source code for the implement an abstract class PlayerBaseState:
using UnityEngine;
public abstract class PlayerBaseState
{
public abstract void EnterState(PlayerController_FSM player);
public abstract void Update(PlayerController_FSM player);
public abstract void OnCollisionEnter(PlayerController_FSM player);
}
Create a new C# script named PlayerIdleState.
The script needs to be changed into this source code:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerIdleState : PlayerBaseState
{
}
Select the PlayerIdleState and use these keys: Cmd or Ctrl and . (the point key) .This will show a menu named Generate overrides... and help the developer to generate the new code:
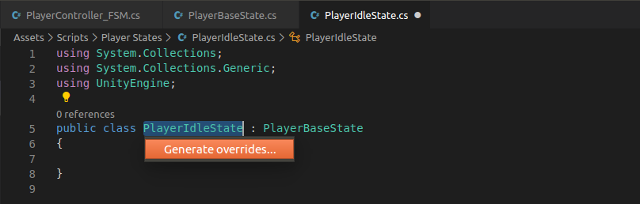
The result is this source code with all new override for each of methods of PlayerBaseState class:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerIdleState : PlayerBaseState
{
public override void EnterState(PlayerController_FSM player)
{
throw new System.NotImplementedException();
}
public override bool Equals(object obj)
{
return base.Equals(obj);
}
public override int GetHashCode()
{
return base.GetHashCode();
}
public override void OnCollisionEnter(PlayerController_FSM player)
{
throw new System.NotImplementedException();
}
public override string ToString()
{
return base.ToString();
}
public override void Update(PlayerController_FSM player)
{
throw new System.NotImplementedException();
}
}