Google announced that Android 16(stable) will be available to all Pixel devices by tomorrow starting with Pixel 9 series
2D, 3D, game, games, online game, game development, game engine, programming, OpenGL, Open AI, math, graphics, design, graphic, graphics, game development, game engine, programming, web development, web art, web graphic, arts, tutorial, tutorials,
Se afișează postările cu eticheta android. Afișați toate postările
Se afișează postările cu eticheta android. Afișați toate postările
luni, 9 iunie 2025
TickTick :To Do List & Calendar for management time application.
I don't just use one time management application on my phone. The main reason is that there isn't a perfect one, and moreover, almost all of them cost quite a bit. If I were to add up all the costs, they wouldn't justify themselves. Today I am going to present one that is quite useful, which I discovered can send notifications on the screen to check off the daily steps to follow, and additionally, it also has a centralized widget.
You can find this android application on the google play store.

vineri, 11 aprilie 2025
News : Calendar Ortodox PATRIARHIA ROMÂNĂ - android application.
The android application with the Orthodox calendar of the Romanian Patriarchate was launched this year and you can find it at google play - Calendar Ortodox PATRIARHIA ROMÂNĂ.


joi, 3 aprilie 2025
luni, 6 ianuarie 2025
News : Two lumalabs creation model and 3D capture.
The create feature from prompt can be tested on this webpage.
The application for 3D capture can be found on android play or apple store, and cam be update on web , see:
Posted by
Cătălin George Feștilă
Labels:
2025,
2025 news,
android,
artificial intelligence,
lumalabs.ai

joi, 3 octombrie 2024
Pydroid 3 android application for python ...
The Pydroid3 android application is the best way to test and run python scripts.
I install python packages with the install option, the pip command on terminal comes with errors for same python package.
I used the free version but if you pay for monthly or lifetime then you can have more features.
Same team come with android applications to have add more features, see these IIEC team appplicatons.




sâmbătă, 31 august 2024
News : Shaders | Jetpack Compose Tips
... about shaders fron the official youtube channel : AndroidDevelopers.
Posted by
Cătălin George Feștilă
Labels:
2024,
2024 news,
android,
news,
tutorial,
tutorials,
video,
video tutorial,
youtube

joi, 29 august 2024
News : Walkthrough Series: Interaction Settings.
... I find a good application on android play to create many types of files SVG files. You can use SVG files in Godot game engine.
Posted by
Cătălin George Feștilă
Labels:
2024,
2024 news,
2D,
android,
Concepts App,
Godot,
graphic,
graphics,
news

vineri, 7 iunie 2024
sâmbătă, 23 martie 2024
News : Android applications on windows 11.
... 2D and 3D graphics processing and interfaces have a different twist, Windows 11 and Android.
marți, 12 martie 2024
Free - some useful Android apps that I use.
These are some android applications that I use and that I consider useful and pleasant to use.
You can find all of these on my google sites webpage.
sâmbătă, 9 martie 2024
News : Online tool for JavaScript and TypeScript
Using this online tool you can create a JavaScript/TypeScript project that runs natively on all your users' devices: web, android, iOS.
You can find more details and the documentation on the official page with the documentation.
An very old video example from 7 years ago, see the official youtube.
Here is a screenshot of this online tool I tested today:
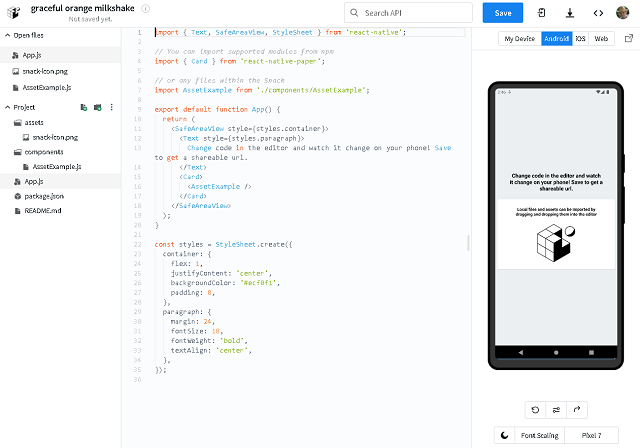
Posted by
Cătălin George Feștilă
Labels:
2024,
2024 news,
android,
iOS,
javascript,
news,
online tool,
React Native,
tutorial,
tutorials,
typescript,
video,
video tutorial,
web,
web development

joi, 15 februarie 2024
Shader Editor - example 005.
Today I posted on my instagram three shaders created to be used as phone wallpapers using the Shader Editor Android software option.
Somewhere in my posts I specified that this android application allows you to create shaders and then you can add them as dynamic wallpaper on phone.
The three source codes are open-source and you can test and use them with the android application and they will run very well as wallpapers:
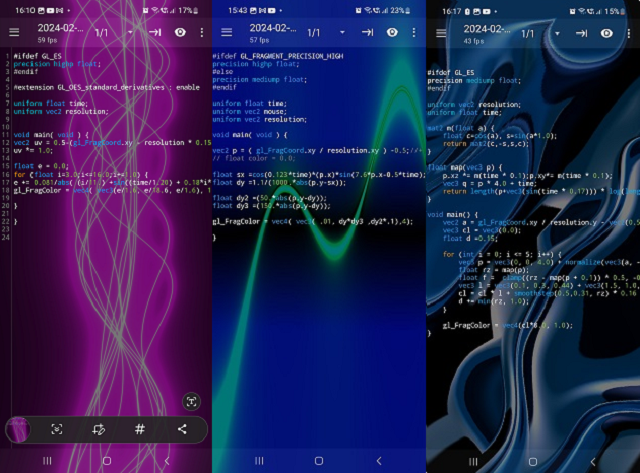
This is a shader that I like as a design because it is relaxing and I put a more feminine color because spring is coming.
#ifdef GL_ES precision highp float; #endif #extension GL_OES_standard_derivatives : enable uniform float time; uniform vec2 resolution; void main( void ) { vec2 uv = 0.5-(gl_FragCoord.xy - resolution * 0.15) / max(resolution.x, resolution.y) * 5.0; uv *= 1.0; float e = 0.0; for (float i=3.0;i<=16.0;i+=1.0) { e += 0.081/abs( (i/11.) +sin((time/1.20) + 0.18*i*(uv.y) *( cos(i/2.10 + (time / 11.0) - uv.y*.4) ) ) + 2.5*uv.x); gl_FragColor = vec4( vec3(e/1.6, e/18.6, e/1.6), 1.0); } }
This is a dynamic sin cos combination
#ifdef GL_FRAGMENT_PRECISION_HIGH precision highp float; #else precision mediump float; #endif uniform float time; uniform vec2 mouse; uniform vec2 resolution; void main( void ) { vec2 p = ( gl_FragCoord.xy / resolution.xy ) -0.5;//+ mouse / 4.0; // float color = 0.0; float sx =cos(0.123*time)*(p.x)*sin(7.6*p.x-0.5*time); float dy =1.1/(1000.*abs(p.y-sx)); float dy2 =(50.*abs(p.y-dy)); float dy3 =(150.*abs(p.y-dy)); gl_FragColor = vec4( vec3( .01, dy*dy3 ,dy2*.1),4); }
This shader has a darker style:
#ifdef GL_ES precision mediump float; #endif uniform vec2 resolution; uniform float time; mat2 m(float a) { float c=cos(a), s=sin(a*1.0); return mat2(c,-s,s,c); } float map(vec3 p) { p.xz *= m(time * 0.1);p.xy*= m(time * 0.1); vec3 q = p * 4.0 + time; return length(p+vec3(sin(time * 0.17))) * log(length(p) + 0.10) + sin(q.x + sin(q.z + sin(q.y))) * 1.8; } void main() { vec2 a = gl_FragCoord.xy / resolution.y - vec2(0.5, 0.5); vec3 cl = vec3(0.0); float d =0.15; for (int i = 0; i <= 5; i++) { vec3 p = vec3(0, 0, 4.0) + normalize(vec3(a, -1.0)) * d; float rz = map(p); float f = clamp((rz - map(p + 0.1)) * 0.5, -0.1, 1.0); vec3 l = vec3(0.1, 0.3, 0.44) + vec3(1.5, 1.0, 1.0) * f; cl = cl * l + smoothstep(0.5,0.31, rz) * 0.16 * l; d += min(rz, 1.0); } gl_FragColor = vec4(cl*8.0, 1.0); }
Posted by
Cătălin George Feștilă
Labels:
2024,
3D,
3d software,
android,
shader,
Shader Editor,
tutorial,
tutorials

vineri, 12 ianuarie 2024
The Ren'Py visual novel engine .
Ren'Py is a visual novel engine – used by thousands of creators from around the world – that helps you use words, images, and sounds to tell interactive stories that run on computers and mobile devices. These can be both visual novels and life simulation games. The easy to learn script language allows anyone to efficiently write large visual novels, while its Python scripting is enough for complex simulation games.
Ren'Py is free to use with commercial and non-commercial games and you can find on the official website.
This run's on Android 5.0+,HTML5/Web Assembly (Beta),Linux x86_64/Arm,Windows 7+,Mac OS X 10.10+ and iOS 11+.
This is last video tutorial from RenPy start tutorials but you can find more on web.
Posted by
Cătălin George Feștilă
Labels:
2024,
2D,
android,
development,
game development,
game engine,
html5,
iOS,
linux,
Mac OS,
RenPy,
web development,
Windows OS

joi, 28 decembrie 2023
News : Online tool for assembly with simulator and debugger ...
CPUlator is a full-system Nios II, ARMv7, and SPIM-compatible MIPS simulator that runs in a web browser. It is designed for education use to teach computer ...
This runs inside a web browser.
- Instruction sets: Nios II, ARMv7, and MIPS
- I/O devices:
- Nios II and ARMv7: Includes most devices found on a DE1-SoC (and other board models used by the Altera University Program), including interrupt support.
- MIPS: Includes SPIM-compatible terminal
- Nothing to install: Runs entirely inside a web browser
- Debugger: Single-step, breakpoints, watchpoints, trace, call stack, examine disassembly, memory and registers
- Debug assertions: Optional runtime assertions catch many potential errors
- Input: Accepts both assembly source code and ELF executables
I tested with the simple basic assemnbly source code and works well:
.global _start
_start:
mov r0,#1
mov r1,#3
push {r0,r1}
bl get_value
pop {r0,r1}
B end
get_value:
mov r0,#5
mov r1,#7
add r2,r0,r1
bx lr
end:
You can save, load and compile this sorce code like a simple file type : simple.s
This is the result:
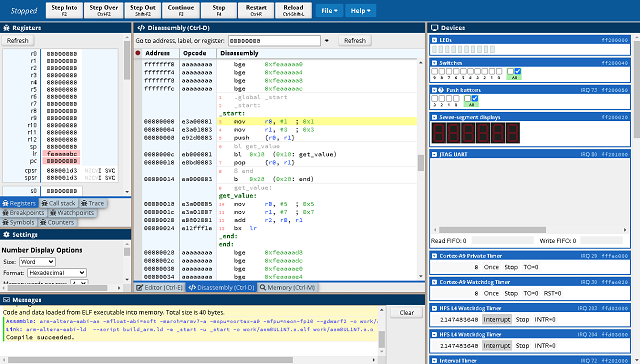
Posted by
Cătălin George Feștilă
Labels:
2023,
2023 news,
android,
assembly,
news,
online,
online tool

miercuri, 9 august 2023
Shader Editor - example 004.
Another example with the Shader Editor android application, this time simpler:
This shader displays some concentric circles that change their colors according to a sine and cosine formula.
This is the source code for the shader:
#ifdef GL_FRAGMENT_PRECISION_HIGH
precision highp float;
#else
precision mediump float;
#endif
uniform vec2 resolution;
uniform float time;
void main(void) {
float mx = max(resolution.x, resolution.y);
vec2 uv = gl_FragCoord.xy / mx;
vec2 center = resolution / mx * 0.5;
float t = time * 10.0;
gl_FragColor = vec4(cos(t+distance(uv, center) / 222.0)+1.0,
vec2(sin(t - distance(uv, center) * 76.0)) * 10.5,
1.0);
}
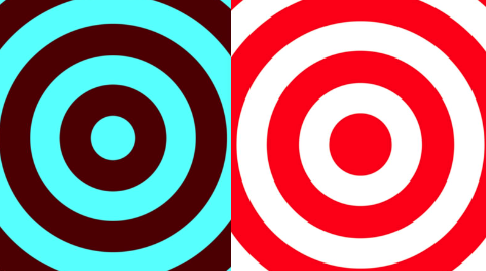
Posted by
Cătălin George Feștilă
Labels:
2023,
3D,
3d software,
android,
shader,
Shader Editor,
tutorial,
tutorials

marți, 8 noiembrie 2022
Shader Editor - example 003.
Today I will show you how to change a shader from shadertoy website to a shader for Shader Editor android aplication.
Open my shader example from shader toy website.
#define f length(fract(q*=m*=.6+.1*d++)-.5)
void mainImage( out vec4 fragColor, in vec2 fragCoord )
{
float d = 0.;
//vec3 uv = vec3(fragCoord.xy / iResolution.xy,iTime*.2);
vec3 q = vec3(fragCoord.xy/iResolution.yy-13., iTime*.2);
mat3 m = mat3(-2,-1,2, 3,-2,1, -1,1,3);
vec3 col = vec3(pow(min(min(f,f),f), 7.)*40.);
fragColor = vec4(clamp(col + vec3(0., 0.35, 0.5), 0.0, 1.0), 1.0);
}
In order to use it in the Shader Editor android application you need to make these changes:
#ifdef GL_FRAGMENT_PRECISION_HIGH
precision highp float;
#else
precision mediump float;
#endif
uniform vec2 resolution;
uniform float time;
uniform vec4 mouse;
uniform vec4 date;
#define f length(fract(q*=m*=.6+.01*d++)-.5)
void mainImage( out vec4 fragColor, in vec2 fragCoord )
{
float d = 0.91;
vec3 q = vec3(fragCoord.xy/resolution.yy-13., time*.2);
mat3 m = mat3(-2,-3,6, 3,-1,1, 0,3,1);
vec3 col = vec3(pow(min(min(f,f),f), 7.)*40.);
fragColor = vec4(clamp(col + vec3(0., 0.35, 0.5), 0.0, 1.0), 1.0);
}
void main() {
vec4 fragment_color;
mainImage(fragment_color, gl_FragCoord.xy);
gl_FragColor = fragment_color;
}
You can see these changes are n the first part of the source code and just one on time.
You can play with this source code then use the option Set as wallpaper to have it on your phone.
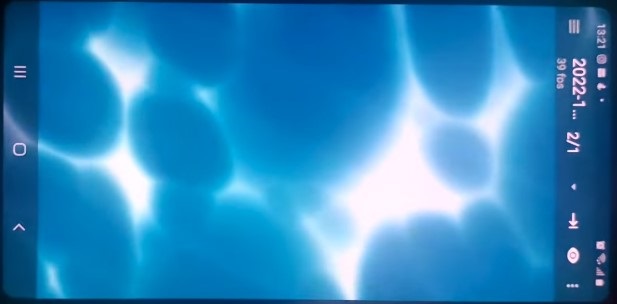
Posted by
Cătălin George Feștilă
Labels:
2022,
3D,
3d software,
android,
shader,
Shader Editor,
tutorial,
tutorials

marți, 1 noiembrie 2022
Shader Editor - example 002.
This post is just one simple example, you can find many tutorials if you search on web for my work ...
If you don't have enough time to test my last shader source code then you can test now with another source code to have a beautiful blue effect.

Let's see the source code for this shader:
#ifdef GL_FRAGMENT_PRECISION_HIGH
precision highp float;
#else
precision mediump float;
#endif
uniform vec2 resolution;
uniform float time;
uniform vec4 mouse;
uniform vec4 date;
//#define time iTime
#define R resolution.xy
void mainImage( out vec4 fragC, in vec2 fC)
{
// vec2 pos = (fragCoord.xy/R.xy) * 8. - 4.;
vec2 pos = (fC.xy/R.xy) * 8. - 4.;
pos.x *= R.x / R.y;
float s = .25, f = .0, k = f;
vec3 p = vec3(pos, sin(time * .4) * .5 - .5)* s;
for( int i=0; i< 9; i++ )
{
p = abs(p)/dot(p,p)- 1.5;
k = length(p) ;
p = p*k+k;
}
f = dot(p,p)* s;
fragC= vec4(f*.5, f *1.2, f * 5., 0.111);;
}
void main() {
vec4 fragment_color;
mainImage(fragment_color, gl_FragCoord.xy);
gl_FragColor = fragment_color;
}
Posted by
Cătălin George Feștilă
Labels:
2022,
3D,
3d software,
android,
shader,
Shader Editor,
tutorial,
tutorials

sâmbătă, 29 octombrie 2022
Shader Editor - example 001.
You can test your skills in shader theory with this excellent android application named Shader Editor.
The application can be found on google play.
After installation, you can use the eye icon to toggle between the source code and the output and the three dots icon to load, load samples, and save ...
If you want to rename it after creating a new shader or save it as a new shader, just click on the icon with three lines and double-click on the shader's name.
This is a shader created for my cardboard virtual reality and set for my eye anatomy ...
#ifdef GL_FRAGMENT_PRECISION_HIGH
precision highp float;
#else
precision mediump float;
#endif
uniform vec2 resolution;
uniform vec2 cameraAddent;
uniform mat2 cameraOrientation;
uniform samplerExternalOES cameraBack;
void main(void) {
vec2 uv = gl_FragCoord.xy / resolution.xy;
vec2 st = cameraAddent + uv* cameraOrientation * vec2(tan(0.805),cos(0.31));
gl_FragColor= vec4(
texture2D(cameraBack, st*vec2(2.0,1.0)).rgb,0.1);
}
Posted by
Cătălin George Feștilă
Labels:
2022,
3D,
3d software,
android,
shader,
Shader Editor,
tutorial,
tutorials

joi, 10 martie 2022
Modding State of Survival with BlueStacks X.
BlueStacks X is the world's first cloud-based Android gaming service that lets you play games on any of your devices, without having to download them.
The game modding the short for modification, is the process of alteration by players or fans of one or more aspects of a video game, such as how it looks or behaves, and is a sub-discipline of general modding.
State of Survival is a zombie themed mobile game that requires players' team work and strategic thinking to survive in a post-apocalyptic world overrun with zombies.
You can see the game on this webpage.
Posted by
Cătălin George Feștilă
Labels:
2022,
2D,
3D,
android,
FunPlus International,
Game,
games,
modding

Abonați-vă la:
Postări (Atom)